How to develop Scalable Programs to be a Developer By Gustavo Woltmann
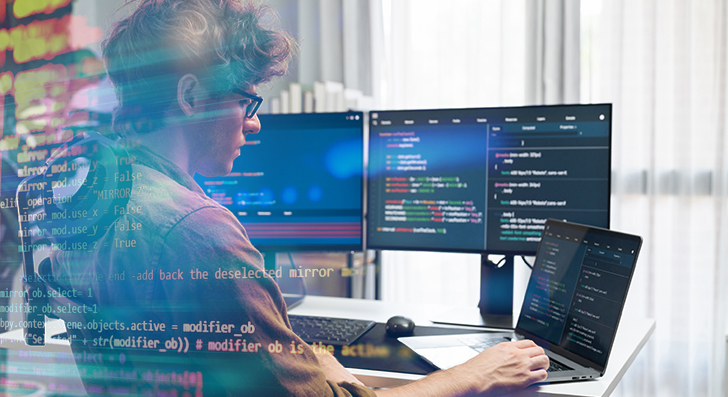
Scalability implies your software can deal with growth—extra end users, a lot more data, and more website traffic—with no breaking. As being a developer, creating with scalability in mind will save time and stress afterwards. Right here’s a transparent and functional guide to assist you to get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Many apps fail whenever they grow rapidly because the initial design can’t take care of the extra load. Being a developer, you need to Consider early regarding how your method will behave stressed.
Start by planning your architecture to generally be flexible. Avoid monolithic codebases in which all the things is tightly connected. Alternatively, use modular structure or microservices. These patterns break your app into scaled-down, independent areas. Each individual module or services can scale on its own devoid of influencing the whole method.
Also, think of your databases from day a single. Will it need to have to take care of one million users or perhaps a hundred? Select the appropriate style—relational or NoSQL—depending on how your knowledge will improve. Approach for sharding, indexing, and backups early, Even when you don’t need them still.
Yet another critical place is to stop hardcoding assumptions. Don’t compose code that only performs beneath recent ailments. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like concept queues or function-driven techniques. These aid your app take care of far more requests with no acquiring overloaded.
Once you Construct with scalability in mind, you are not just making ready for achievement—you are decreasing foreseeable future complications. A effectively-planned system is less complicated to keep up, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the ideal Databases
Selecting the right databases is actually a important part of making scalable apps. Not all databases are constructed the same, and using the Erroneous one can gradual you down or maybe result in failures as your application grows.
Start out by knowing your data. Could it be extremely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient fit. These are definitely sturdy with relationships, transactions, and consistency. In addition they assist scaling strategies like read replicas, indexing, and partitioning to manage more targeted traffic and information.
If the information is much more flexible—like consumer exercise logs, merchandise catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured facts and can scale horizontally far more conveniently.
Also, contemplate your read and publish styles. Are you currently undertaking lots of reads with fewer writes? Use caching and browse replicas. Will you be handling a large produce load? Explore databases that could tackle higher compose throughput, or maybe party-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Imagine ahead. You may not require Innovative scaling capabilities now, but deciding on a databases that supports them means you won’t need to switch later.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your details depending on your access patterns. And always keep track of database overall performance as you grow.
In a nutshell, the best databases is dependent upon your app’s construction, speed requirements, and how you expect it to grow. Take time to select wisely—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Badly created code or unoptimized queries can decelerate general performance and overload your process. That’s why it’s crucial that you Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything needless. Don’t choose the most elaborate Resolution if a simple a person performs. Keep your capabilities quick, focused, and simple to check. Use profiling tools to uncover bottlenecks—areas where your code can take also long to operate or utilizes far too much memory.
Up coming, look at your database queries. These usually gradual items down much more than the code by itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and alternatively select distinct fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout large tables.
In case you see the identical facts getting asked for many times, use caching. Retailer the final results quickly utilizing equipment like Redis or Memcached this means you don’t have to repeat pricey functions.
Also, batch your databases functions when you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more successful.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred data could possibly crash after they have to manage one million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with much more consumers and even more targeted traffic. If almost everything goes by way more info of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two equipment aid keep your application speedy, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the get the job done, the load balancer routes end users to distinctive servers based upon availability. What this means is no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this straightforward to build.
Caching is about storing info temporarily so it can be reused promptly. When end users request the exact same data once more—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for fast entry.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things which don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are simple but effective resources. Jointly, they assist your app take care of extra customers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you require resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They provide you adaptability, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you can add much more sources with just a few clicks or immediately utilizing automobile-scaling. When visitors drops, you are able to scale down to save money.
These platforms also provide providers like managed databases, storage, load balancing, and safety resources. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are A different critical Device. A container deals your app and all the things it ought to operate—code, libraries, settings—into one device. This can make it uncomplicated to move your app concerning environments, from the laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of one's application crashes, it restarts it routinely.
Containers also allow it to be straightforward to independent parts of your application into solutions. You could update or scale areas independently, which is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and Get well quickly when troubles happen. If you need your application to expand without the need of limitations, start out utilizing these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t keep track of your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and reaction time. These show you how your servers and services are performing. Equipment like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just observe your servers—monitor your app as well. Keep an eye on how long it will take for consumers to load webpages, how often problems take place, and the place they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Build alerts for significant challenges. One example is, If the reaction time goes previously mentioned a limit or even a support goes down, you need to get notified instantly. This helps you fix challenges speedy, generally ahead of end users even recognize.
Monitoring is usually handy if you make adjustments. In the event you deploy a new feature and find out a spike in problems or slowdowns, you'll be able to roll it back in advance of it brings about genuine destruction.
As your application grows, site visitors and data raise. Without having monitoring, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application reputable and scalable. It’s not just about recognizing failures—it’s about comprehending your process and ensuring it really works nicely, even stressed.
Final Feelings
Scalability isn’t only for massive companies. Even modest applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make applications that expand efficiently without breaking under pressure. Start modest, Imagine large, and Make smart.